3D Glider
Platform: Android
Game Engine: Unity
Code Editor: Visual Studio
3D Glider is a physics strategy game in which you leverage various physical facts such as drag, velocity, thrust, and gravity to fly various courses going through a number of boxes in sequence for a best time attempting to get the top rank on the Google Play Leader board of world record best-times. I added the Google Leader board both for the excitement of competing world wide and to drive enthusiasm. Players are also able to purchase a new skin with an in-app-purchase.
History:
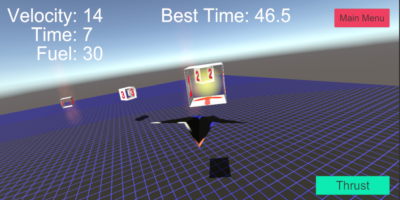
Challenge:
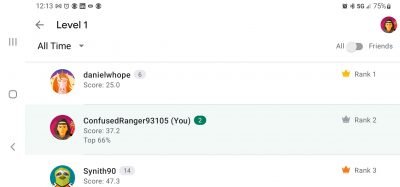
Solution:
At first I considered doing a binary search of my repository to see were the crash started but didn’t have any good idea what to do next beyond progressively removing new code until the builds stopped crashing. I then realized I could use a virtual device and preceded to set up a Virtual Pixel 2. In the process of setting it up I discovered that it had an old Android version which I suspected was crashing. I also realized that the new Google Play requirement was now a higher level. I adjusted the required SDK, resubmitted an Internal test and was happy to see that it was no longer crashing.
Challenge:
I ran into another issue when setting up in-app purchases. I was logging into Google from the Main Menu scene but when I debug I would run the Level scene and so the store was not being initialized.
Solution:
I solved this issue by creating a singleton game manager and adding it to each scene I may want to start from:
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using GooglePlayGames;
using GooglePlayGames.BasicApi;
using TMPro;
public class GameManager : MonoBehaviour
{
private static GameManager _instance;
[SerializeField] private GameObject messageLogScrollView;
[SerializeField] private TextMeshProUGUI message;
private Toggle debugModeToggle;
public static GameManager Instance
{
get
{
if(_instance==null)
{
Debug.Log("GameManager.Instance is NULL");
}
return _instance;
}
}
void OnEnable()
{
Application.logMessageReceived += LogCallback;
SetShowMessageLogActive(GamePlayerPrefs.debugFlag);
}
private void Awake()
{
if(_instance == null)
{
_instance = this;
}
else
{
DestroyImmediate(gameObject);
}
PlayGamesPlatform.DebugLogEnabled = true;
PlayGamesPlatform.Activate();
}
//Called when there is an exception
void LogCallback(string condition, string stackTrace, LogType type)
{
if(type!=LogType.Log)
{
message.text += $"Type: {type}\n\n\nCondition: {condition}\n\n\nStack Trace: {stackTrace}\n\n\n";
if(debugModeToggle)
{
debugModeToggle.isOn = true;
}
else
{
GamePlayerPrefs.debugFlag = false;
}
}
}
void OnDisable()
{
Application.logMessageReceived -= LogCallback;
}
private void Start()
{
var debugPanelGO = GameObject.FindObjectOfType();
if(debugPanelGO)
{
debugModeToggle = debugPanelGO.gameObject.GetComponent();
LoginToGoogle();
if(gameObject.transform.parent==null)
{
DontDestroyOnLoad(gameObject);
}
}
}
static public void SetShowMessageLogActive(bool flag)
{
Instance?.messageLogScrollView.SetActive(flag);
}
private void LoginToGoogle()
{
PlayGamesPlatform.Instance.Authenticate(ProcessAuthentification);
}
private void ProcessAuthentification(SignInStatus status)
{
if(SignInStatus.Success == status)
{
message.text = $"Authentification: {status}\n\n\n";
}
else
{
message.text = $"Authentification: {status}\n\n\n";
}
if(false)
{
StartCoroutine(ExecuteAfterTime(2.0f, () =>
{
ClearAutentificationMessage();
}));
}
}
private bool isCoroutineExecuting = false;
IEnumerator ExecuteAfterTime(float time, Action task)
{
if(isCoroutineExecuting)
yield break;
isCoroutineExecuting = true;
yield return new WaitForSeconds(time);
task();
isCoroutineExecuting = false;
}
void ClearAutentificationMessage()
{
message.text = "";
}
}